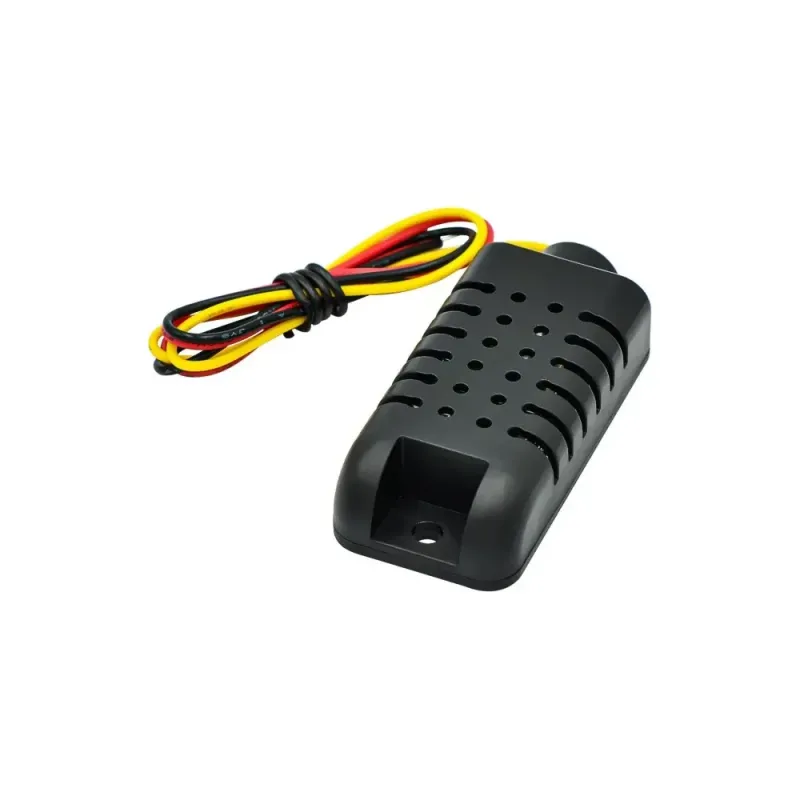
- Stock: In Stock
- Model: SNC004.AM2301A
AM2301A Digital Temperature Humidity Sensor Module
The AM2301A, also referred to as DHT21, is a digital sensor designed for measuring temperature and humidity. The following details provide an overview of the sensor's specifications and functionality:
Key Features of the AM2301A Sensor:
- Temperature Measurement:
- Accuracy: ±0.3°C
- Measurement Range: -40 to 125°C
- Resolution: 0.1°C
- Humidity Measurement:
- Accuracy: ±2% RH
- Measurement Range: 0 to 100% RH
- Resolution: 0.1% RH
- Power Supply: 3.3V to 5.5V DC
- Output: Digital signal (single-wire communication protocol)
- Dimensions: Typically around 15mm x 12mm x 5.5mm
- Response Time: Typically less than 1 second
- Integration: Easily interfaces with microcontrollers like Arduino, Raspberry Pi, etc.
Feature Descriptions:
The AM2301A offers ±0.3°C temperature precision and ±2% RH humidity precision, making it a reliable choice for accurate readings. It operates over a broad temperature range from -40 to 125°C, providing versatility in various use cases. The sensor is capable of measuring humidity from 0% to 100% RH with high precision and resolution. It supports a wide range of power supply voltages, ensuring compatibility with various systems and applications. The sensor responds quickly to changes, typically in less than 1 second, making it ideal for applications that require fast data collection.
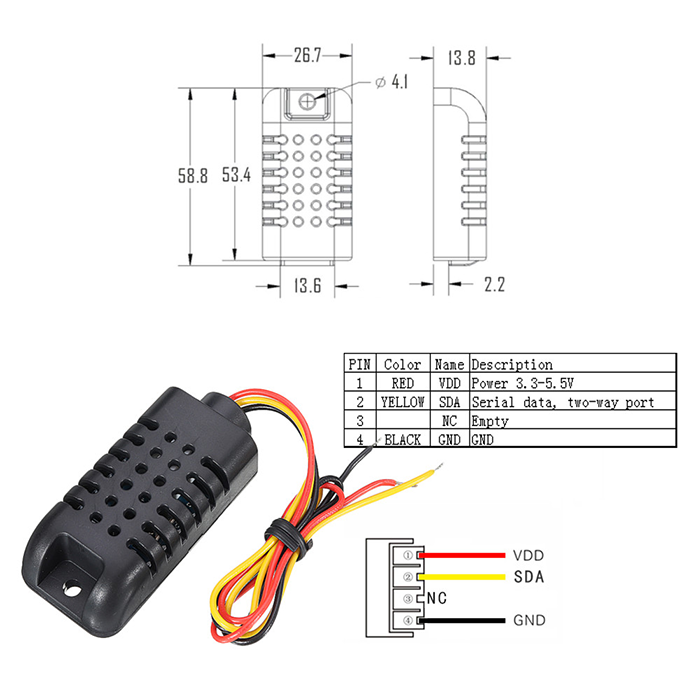
Applications:
The AM2301A sensor is frequently used in weather monitoring, HVAC systems, industrial automation, and consumer electronics to monitor temperature and humidity levels. It delivers reliable performance with high precision and low power consumption, making it suitable for a wide range of applications.
Example Arduino Code:
The following is a basic example of how to use the AM2301A (DHT21) sensor with an Arduino:
#include
#define DHTPIN 2 // Digital pin connected to the sensor
#define DHTTYPE DHT21 // AM2301 (DHT21) sensor type
DHT dht(DHTPIN, DHTTYPE);
void setup() {
Serial.begin(9600);
dht.begin();
}
void loop() {
delay(2000); // Delay between readings
float temperature = dht.readTemperature(); // Read temperature in Celsius
float humidity = dht.readHumidity(); // Read humidity
// Check if any reads failed and exit early (to try again)
if (isnan(temperature) || isnan(humidity)) {
Serial.println("Failed to read from sensor!");
return;
}
Serial.print("Temperature: ");
Serial.print(temperature);
Serial.print(" °C Humidity: ");
Serial.print(humidity);
Serial.println(" %");
}
This code initializes the DHT library, reads temperature and humidity data from the AM2301A sensor connected to pin 2, and displays the values on the Serial Monitor every 2 seconds. Adjust the DHTPIN and DHTTYPE as needed based on your setup.